Are you stuck at the concept of how will you free the allocated memory in C programming. Don’t worry, we will provide a full guidance regarding the functions of memory allocation in c language.
In C programming, we use some fixed rules because it is a structured language. In all its rules and functions, it also involves altering the array size (an array is a collection of items stored at a contiguous memory location). Let us understand it with the help of an example of array before discussing the procedure of how will you free the allocated memory.
Elements | 20 | 65 | 24 | 17 | 26 | 98 | 68 | 43 |
Array indices | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
Here-
Number of elements=8
Array length=8
First Index=0
Last Index=7
Now two situations will occur here-
- If we have array length 10, and we need only 8 elements to enter then in this case 2 array blocks will be wasted. So we need to lessen the size of the array upto 8.
- Second case is if the size of the array is 7 and we have more than 7 elements to insert. Consider we have to insert 9 elements then there is a need to large the size of the array so that we can insert all the elements.
In both the cases we require to change the length of the array to avoid the wastage of memory or to use memory as the number of elements.
The memory allocated by the operating system for an object (a variable, instances of classes and structure) is called memory allocation. It is a process of allocating blocks of memory in a program that is used to store the variables and examples of structure and classes.
There are two types of memory allocation-
- SMA (Static Memory Allocation)
- DMA (Dynamic Memory Allocation)
Static memory allocation-
Table of Contents
when the variables are declared in the statement, then the compiler allocates memory for the variables at the compile-time. When a decision is made on the compile-time, how much memory is needed to store the elements rather than run time is named compile-time memory allocation or static memory allocation.
As the name suggests, we cannot change the memory allocation of the elements; it is fixed. For example-
main ()
{
int a;
float b;
char c;
int x[5]
}
To put it more simply, let’s say we need to enter 5 elements and the memory size of one element is 2 byte, then the compiler will allocate the 10 bytes memory for the elements.
Dynamic memory allocation-
When the decision is taken on run time,how much memory is needed according to the element, it is called dynamic memory allocation. Suppose if we do not know how many elements we require to enter 1 or 10 or 100? Then we need special functions to adjust the memory.
In C language 4 functions are there that help you on how will you free the allocated memory. After the use of memory it is our responsibility to free the memory when it is not needed. These four library functions are defined in the <stdlib.h> header file. These four functions are-
- Memory allocation function or malloc
- Contiguous allocation function or calloc
- Real allocation function or realloc
- Free or deallocation function
Now we will discuss these four functions and learn how will you free the allocated memory.
1. Memory allocation or malloc-
It is a primarily used method to fix a single large block of memory dynamically with the specified size. Moreover, it returns a void pointer that can be adjusted in any form because it does not deal with the type; it considers only size or one argument we can provide in the malloc function. It can be casted into int, float, and char, etc.
Syntax of malloc ()
ptr(data type*) malloc(size)
malloc(5)- It means one variable of 5 bytes
malloc(4)-It means one variable of 4 bytes.
for example-
We have to enter 5 integer variables each of size 4 bytes, then the size of memory=number of elements*size of integers
ptr= (int*) malloc(5*size of the int)):
then we will get 20 bytes of memory for the elements at the run time. It will initialize the default value-
P[0]=2
p[1]=6
p[2]=10 and so on.
p [0] is the location of the first element.
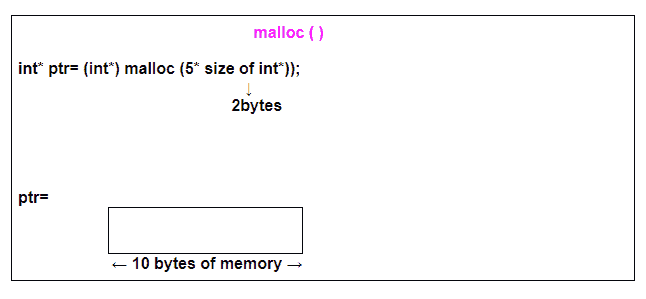
If space is available, allocation will occur, and if space is not available, it will return to null, and allocation fails. So the purpose of malloc function is to allocate memory when we need to enter more elements in an array.

2.contiguous allocation or calloc-
Unlike malloc function, it initializes all the bits to zero when memory is allocated or returns to null if allocation fails. This function is used to allocate the specified number of blocks of memory of the specific type.
Syntax of calloc-
ptr=(data type*) calloc (n,element-size));
For example-
ptr=(float*) calloc (20,size of float));
this command will allocate contiguous space in memory for 20 elements and the size of each element will be in float.
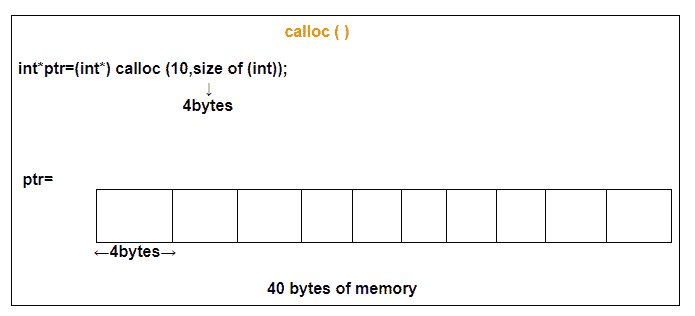
It gives separate blocks for the different elements while in malloc it allocates a single block.
3.Free() method-
This method is the answer to how will you free the allocated memory. In this method, memory blocks are dynamically de-allocated when we do not need them. The memory allocated by the malloc () and calloc () is not de-allocated by themselves,it is our responsibility to release the memory when it is more than enough to avoid wastage of memory.
syntax of Free()-
free (ptr);
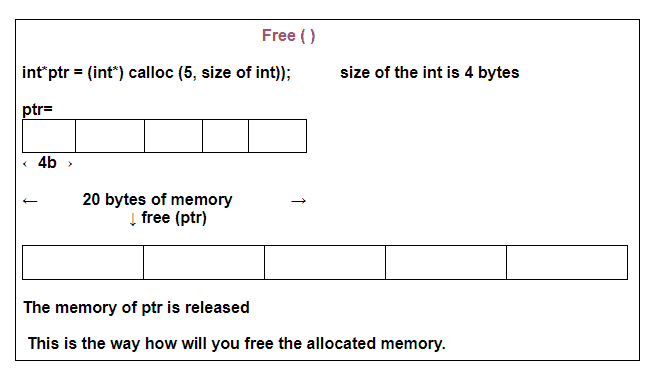
4. Re-allocation method or realloc ( )-
In the programming language C, realloc or re-allocation method is used to change the allocation memory of the previously allocated memory. In simple words, if the memory allocated by the malloc ( ) or calloc ( ) functions is not sufficient then realloc is used to dynamically re-allocate memory. In this method new memory blocks are added.
Syntax-
ptr = realloc (ptr,new size);
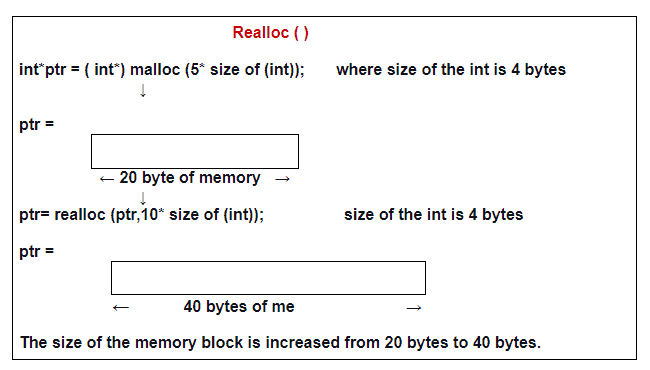
Summary-
In the c language programming after we compile the programme and then run it. Then the data is stored in the memory. But when data is using less memory but the memory blocks are more then there is a need to release the memory so that we can stop the wastage of the memory. The memory will not be free by itself, we have to need some operation or function to release it. (creditcadabra.com) For this purpose we use the free ( ) method in which when we give command in the programme the extra memory will be released after storing the required data. Here we need to insert a <stdlib.h> header file in our program in order to use this method. Moreover, If you need C programming assignment help, you can discuss your requirements with our online experts.